- Documentation >
- Guide >
- Content management >
- Rich Text >
- Create Online Editor plugin
Create custom Online Editor plugin
There are different ways to extend the Online Editor.
Here you can learn how to add your own plugin to the Online Editor.
Follow the procedure below to create a plugin which inserts the current date into
a RichText Field.
Note
Online Editor is based on AlloyEditor, which in turn uses CKEditor.
As a result, you create custom Online Editor plugins in a way similar to
the way you do it in CKEditor.
First, create the plugin file in assets/js/online_editor/plugins/date.js
.
The following code implements an InsertDate
method and attaches it to the editor:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16 | (function (global) {
if (CKEDITOR.plugins.get('date')) {
return;
}
const InsertDate = {
exec: function (editor) {
const now = new Date();
editor.insertHtml( now.toString() );
},
};
global.CKEDITOR.plugins.add('date', {
init: (editor) => editor.addCommand('InsertDate', InsertDate),
});
})(window);
|
Then, modify the Online Editor toolbar by adding a button that inserts the date.
Create a file for the button in assets/js/online_editor/buttons/date.js
:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41 | import PropTypes from 'prop-types';
import AlloyEditor from 'alloyeditor';
import EzButton
from '../../../../vendor/ezsystems/ezplatform-richtext/src/bundle/Resources/public/js/OnlineEditor/buttons/base/ez-button.js';
export default class BtnDate extends EzButton {
static get key() {
return 'date';
}
insertDate(data) {
this.execCommand(data);
}
render() {
const title = 'Date';
return (
<button
className="ae-button ez-btn-ae ez-btn-ae--date"
onClick={this.insertDate.bind(this)}
tabIndex={this.props.tabIndex}
title={title}>
<svg className="ez-icon ez-btn-ae__icon">
<use xlinkHref="/bundles/ezplatformadminui/img/ez-icons.svg#date" />
</svg>
</button>
);
}
}
AlloyEditor.Buttons[BtnDate.key] = AlloyEditor.BtnDate = BtnDate;
eZ.addConfig('ezAlloyEditor.BtnDate', BtnDate);
BtnDate.propTypes = {
command: PropTypes.string,
};
BtnDate.defaultProps = {
command: 'InsertDate',
};
|
Next, enable the plugin and the button.
Add the following code in the webpack.config.js
file, under
// Put your config here
.
This way, Ibexa DXP loads the plugin and button files when loading
the Online Editor:
| eZConfigManager.add({
eZConfig,
entryName: 'ezplatform-richtext-onlineeditor-js',
newItems: [
path.resolve(__dirname, 'assets/js/online_editor/buttons/date.js'),
path.resolve(__dirname, 'assets/js/online_editor/plugins/date.js'),
]
});
|
Finally, add the plugin and its button to your Ibexa DXP configuration:
1
2
3
4
5
6
7
8
9
10
11
12
13 | ezplatform:
system:
admin_group:
fieldtypes:
ezrichtext:
toolbars:
paragraph:
buttons:
date:
priority: 0
ezrichtext:
alloy_editor:
extra_plugins: [date]
|
You have successfully created a custom Online Editor plugin.
You can now run the yarn encore dev
command to regenerate the assets, and create
a Content item with a RichText Field.
The new button appears in the toolbar when editing a Paragraph element and inserts
the current date when clicked:
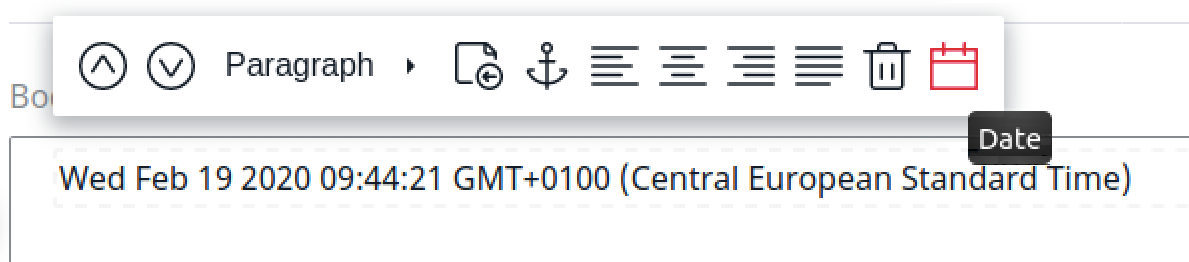